import android.os.Handler
import android.os.Looper
import kotlinx.coroutines.*
import java.util.concurrent.TimeUnit
import kotlin.coroutines.CoroutineContext
object ThreadUtils {
private val ioDispatcher: CoroutineDispatcher = Dispatchers.IO
private val mainDispatcher: CoroutineDispatcher = Dispatchers.Main
private val coroutineScope = CoroutineScope(SupervisorJob() + ioDispatcher)
/**
* 判断是否为主线程
*/
fun isMainThread(): Boolean {
return Looper.getMainLooper().thread == Thread.currentThread()
}
/**
* 后台执行任务
*/
fun execute(runnable: Runnable) {
coroutineScope.launch {
runnable.run()
}
}
/**
* 主线程执行任务
*/
fun executeMain(runnable: Runnable) {
Handler(Looper.getMainLooper()).post(runnable)
}
/**
* 协程版异步执行任务 + 超时控制 + 主线程回调
*/
fun <T> executeWithTimeoutAsync(
timeout: Long,
unit: TimeUnit = TimeUnit.MILLISECONDS,
task: suspend () -> T,
onSuccess: (T) -> Unit,
onError: (Throwable) -> Unit
) {
coroutineScope.launch {
try {
val result = withTimeout(unit.toMillis(timeout)) {
task()
}
withContext(mainDispatcher) {
onSuccess(result)
}
} catch (e: Throwable) {
withContext(mainDispatcher) {
onError(e)
}
}
}
}
}
ThreadUtils.executeWithTimeoutAsync(
timeout = 3,
unit = TimeUnit.SECONDS,
task = {
// 模拟耗时任务
delay(1000)
"结果数据"
},
onSuccess = { result ->
Log.d("ThreadUtils", "成功结果: $result")
},
onError = { error ->
Log.e("ThreadUtils", "出错啦: ${error.message}")
}
)
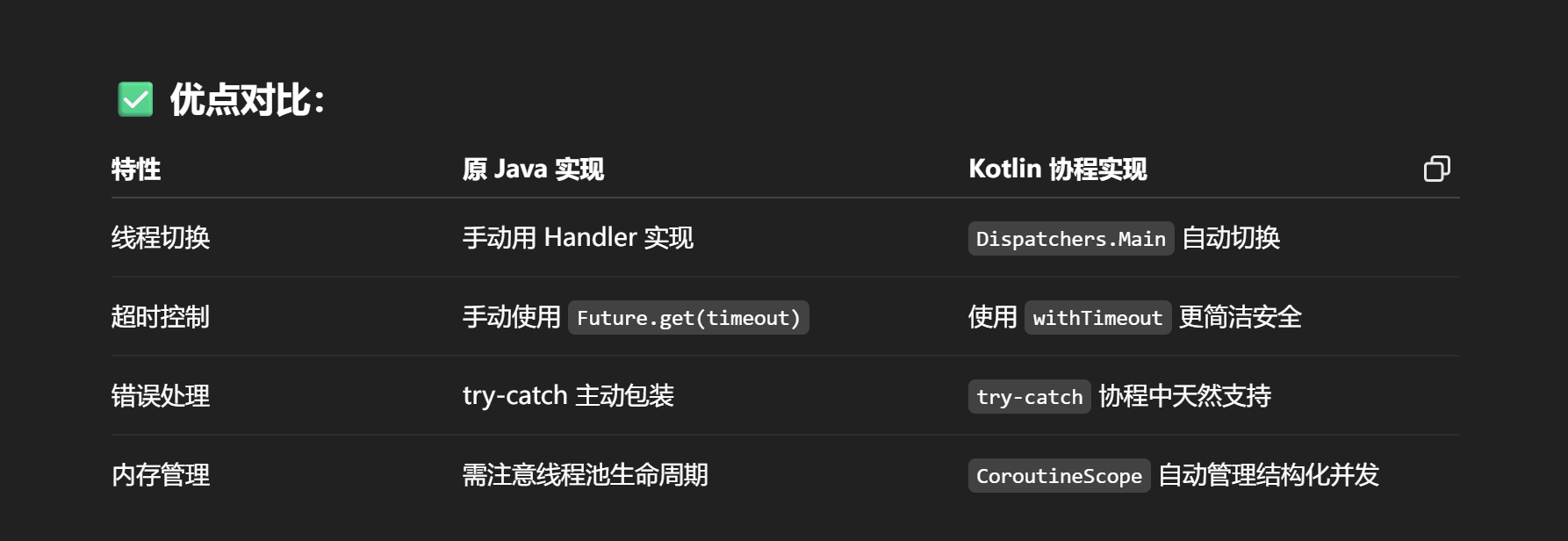