0.RabbitMQ官网通信模式
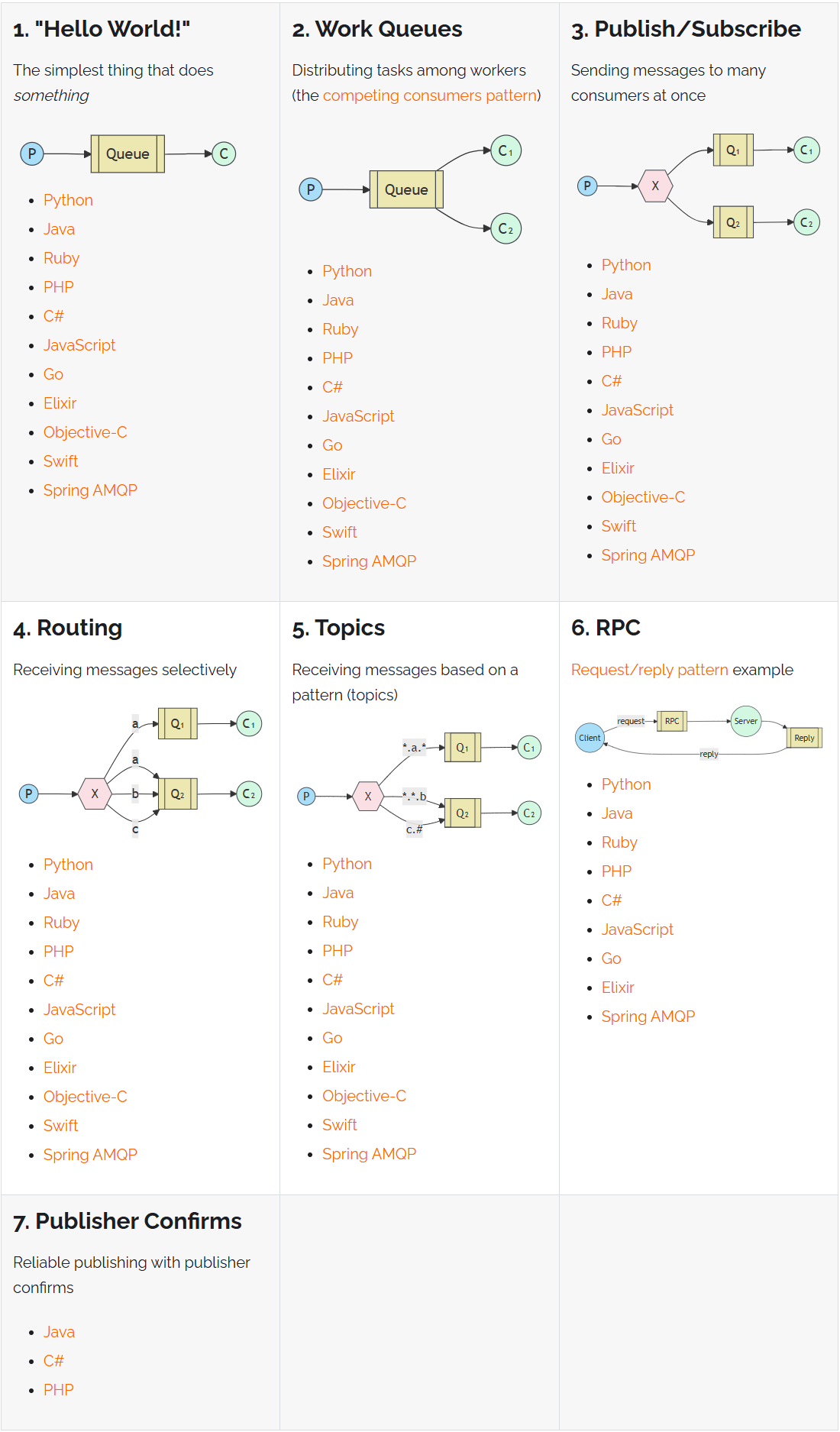
1.Simplest(简单)模式
1.1 发送端
"""
Author: xxx
date: 2025/5/19 11:30
Description:
Simaple简单模式,生产者
简单的RabbitMQ消息队列(不安全,不能持久化)
"""
import pika
rabbitmq_host = '192.168.120.19'
rabbitmq_port = 5672
rabbitmq_username = 'rabbitmq'
rabbitmq_password = 'rabbitmq@123'
credentials = pika.PlainCredentials(rabbitmq_username, rabbitmq_password)
connection = pika.BlockingConnection(
pika.ConnectionParameters(
host=rabbitmq_host,
port=rabbitmq_port,
credentials=credentials
)
)
channel = connection.channel()
queue_name = 'hello'
channel.queue_declare(
queue=queue_name
)
message = 'Hello, World!'
channel.basic_publish(
exchange='',
routing_key=queue_name,
body=message
)
print(" [x] Sent '{}'".format(message))
connection.close()
1.2 接收端
"""
Author: tanggaomeng
date: 2025/5/19 11:44
Description:
Simaple简单模式,消费者
简单的RabbitMQ消息队列(不安全,不能持久化)
"""
import pika
rabbitmq_host = '192.168.120.19'
rabbitmq_port = 5672
rabbitmq_username = 'rabbitmq'
rabbitmq_password = 'rabbitmq@123'
credentials = pika.PlainCredentials(rabbitmq_username, rabbitmq_password)
connection = pika.BlockingConnection(
pika.ConnectionParameters(
host=rabbitmq_host,
port=rabbitmq_port,
credentials=credentials
)
)
channel = connection.channel()
queue_name = 'hello'
channel.queue_declare(
queue=queue_name
)
def callback(ch, method, properties, body):
print(" [x] Received %r" % (body,))
channel.basic_consume(queue=queue_name, on_message_callback=callback, auto_ack=False)
print(' [*] Waiting for messages. To exit press CTRL+C')
channel.start_consuming()
'''
注意:接收到处于死循环,一直在等待接收,发送一个数据,就收到一个数据
'''
基于上面的代码不做任何修改
把上面的消费者开N个就是想要的结果。
如下:
运行3个消费者,生产者生成的消息队列依次被接收者接收,每次只有一个消费者接收到信息,3个消费者接收顺序依次进行
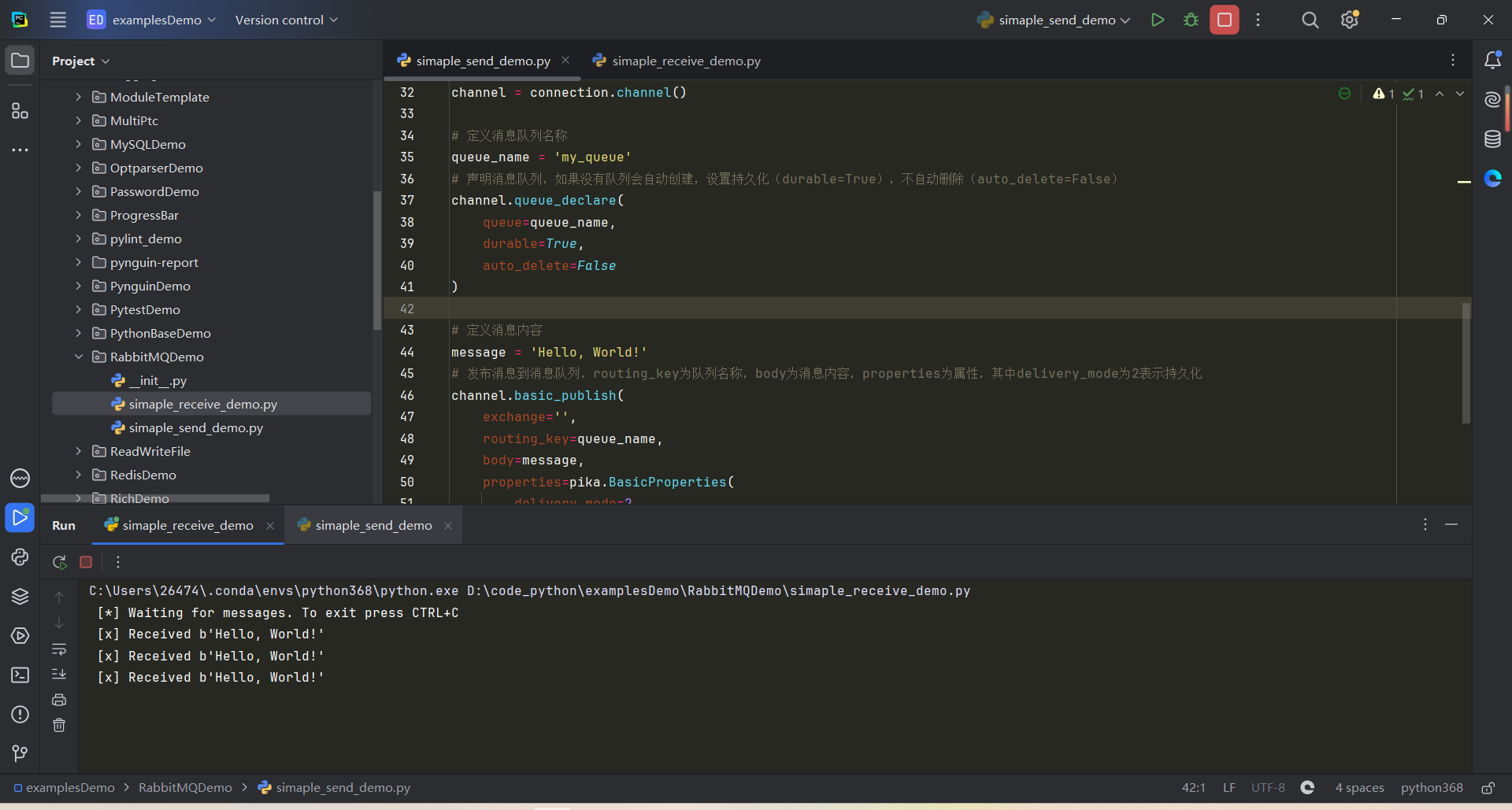
参考:
https://www.rabbitmq.com/tutorials