title: MongoDB索引优化的艺术:从基础原理到性能调优实战
date: 2025/05/21 18:08:22
updated: 2025/05/21 18:08:22
author: cmdragon
excerpt:
MongoDB索引优化与性能调优的核心策略包括:索引基础原理,如单字段、复合、唯一和TTL索引;索引创建与管理,通过FastAPI集成Motor实现;查询性能优化,使用Explain分析、覆盖查询和聚合管道优化;实战案例,如电商平台订单查询优化;常见报错解决方案,如索引创建失败、查询性能下降和文档扫描过多问题。这些策略能显著提升查询速度和系统性能。
categories:
- 后端开发
- FastAPI
tags:
- MongoDB
- 索引优化
- 性能调优
- FastAPI
- 查询分析
- 聚合管道
- 错误处理
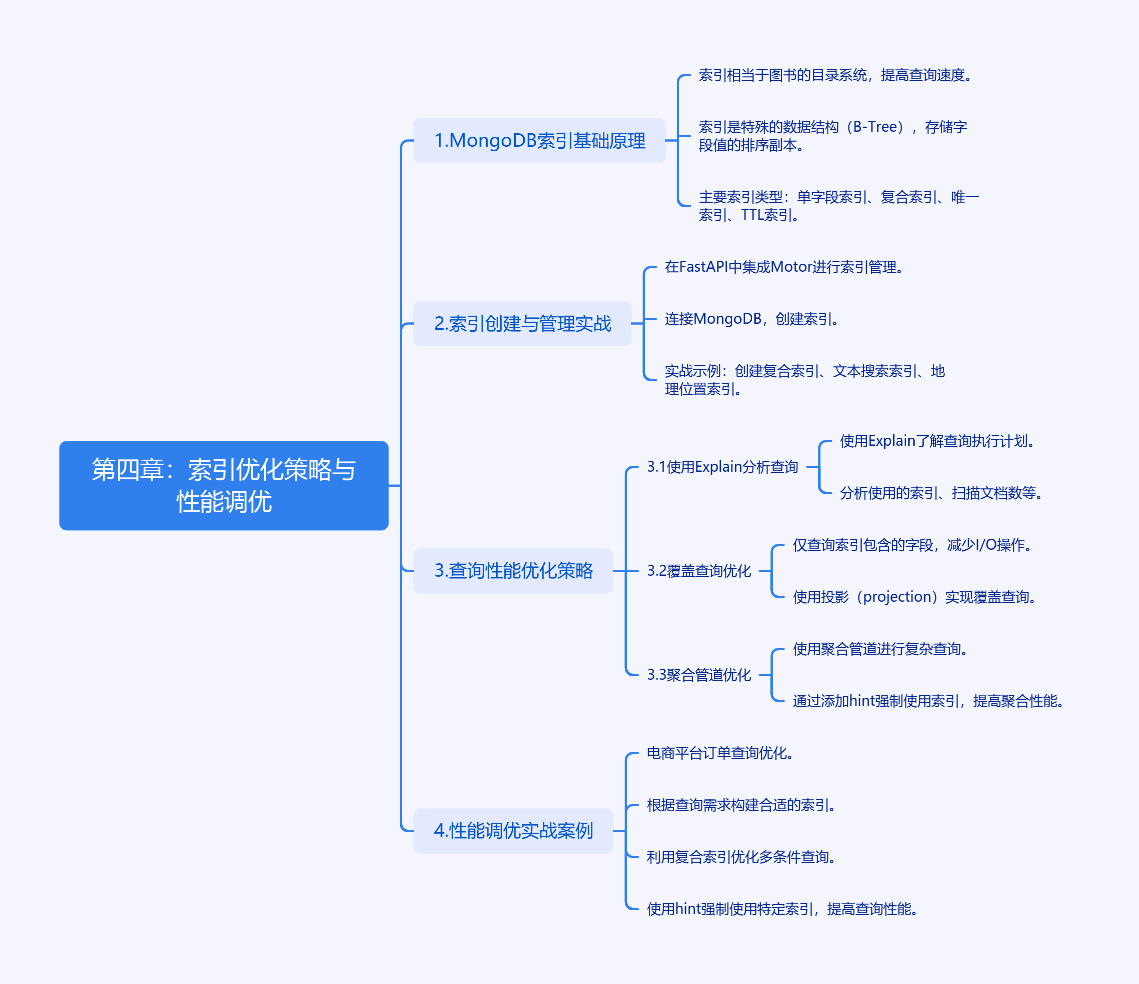
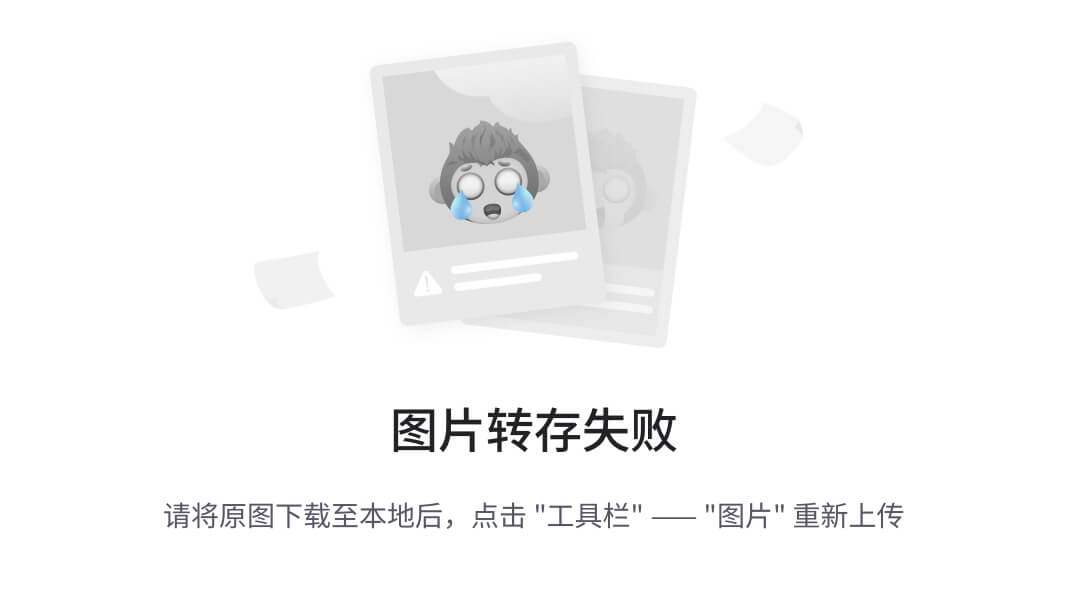
扫描二维码)
关注或者微信搜一搜:编程智域 前端至全栈交流与成长
探索数千个预构建的 AI 应用,开启你的下一个伟大创意:https://tools.cmdragon.cn/
第四章:索引优化策略与性能调优
1. MongoDB索引基础原理
在MongoDB中,索引相当于图书的目录系统。当集合存储量达到百万级时,合理的索引设计能让查询速度提升10-100倍。索引本质上是特殊的数据结构(B-Tree),存储着字段值的排序副本。
主要索引类型:
# 单字段索引示例
async def create_single_index():
await db.products.create_index("name")
# 复合索引示例(注意字段顺序)
async def create_compound_index():
await db.orders.create_index([("user_id", 1), ("order_date", -1)])
# 唯一索引示例
async def create_unique_index():
await db.users.create_index("email", unique=True)
# TTL索引示例(自动过期)
async def create_ttl_index():
await db.logs.create_index("created_at", expireAfterSeconds=3600)
2. 索引创建与管理实战
在FastAPI中集成Motor进行索引管理的最佳实践:
from fastapi import APIRouter
from motor.motor_asyncio import AsyncIOMotorClient
from pydantic import BaseModel
router = APIRouter()
class Product(BaseModel):
name: str
price: float
category: str
# 连接MongoDB
client = AsyncIOMotorClient("mongodb://localhost:27017")
db = client["ecommerce"]
@router.on_event("startup")
async def initialize_indexes